Repository
Current version released
3 years ago
Dependencies
std
Versions
Cabinet, the easier way to manage files in Deno
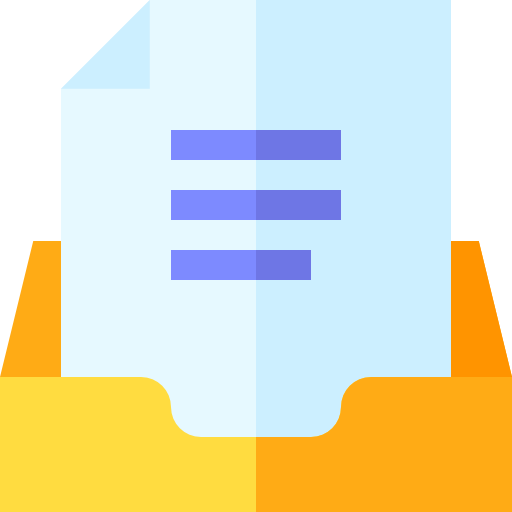
Cabinet
A module created for Deno by DanCodes
Demo
This demo will open a file called testing.txt, write to it and then finally read it and display the contents in the console.
deno run --allow-write --allow-read https://deno.land/x/cabinet/demo.ts
Todo
- Add file moving
- Add resolve function for stringified file
Testing
deno test -A
Use
import {
Cabinet,
CabinetFile,
CabinetError,
} from "https://deno.land/x/cabinet/mod.ts";
const file = new Cabinet("./testing.txt");
file.write("The date is " + new Date());
file.write("The date is " + new Date(), () => console.log("finished!"));
file.writer.sync("This was written synchronously");
file.writer
.promise("This was written with a promise")
.then(() => console.log("finished!"));
file.writer.callback("This was written with a callback", () =>
console.log("finished!")
);
file.read();
file.read(() => console.log("finished!"));
file.reader.sync();
file.reader.promise().then((cFile: CabinetFile) => {
console.log("read " + cFile.size.mb + "mb in promise");
});
file.reader.callback((err?: CabinetError, cFile?: CabinetFile) => {
console.log("read " + cFile?.size.mb + "mb in callback");
});
Format code
deno fmt **/*.ts