Repository
Current version released
2 years ago
Versions
- 2.6.7Latest
- 2.6.6
- 2.6.5
- 2.6.4
- 2.6.3
- 2.6.2
- 2.6.0
- 2.5.5
- 2.5.4
- 2.5.3
- 2.5.2
- 2.5.1
- 2.5.0
- 2.4.11
- 2.4.10
- 2.4.9
- 2.4.8
- 2.4.7
- 2.4.6
- 2.4.5
- 2.4.1
- 2.4.0
- 2.3.5
- 2.3.4
- 2.3.3
- 2.3.2
- 2.3.1
- 2.3.0
- 2.2.3
- 2.2.2
- 2.2.1
- 2.2.0
- 2.1.9
- 2.1.8
- 2.1.7
- 2.1.6
- 2.1.5
- 2.1.1
- 2.0.0
- 1.1.6
- 1.1.5
- 1.1.4
- 1.1.2
- 1.1.0
- 1.0.3
- 1.0.2
- 1.0.1
- 1.0.0
- 0.1.9
- 0.1.8
- 0.1.7
- 0.1.6
- 0.1.5
- 0.1.4
- 0.1.3
- 0.1.2
- 0.1.1
- 0.1.0
- 0.0.5
- 0.0.4
- 0.0.3
- 0.0.2
- 0.0.1
- 0.1
daybreak with WebGPU bindings with desktop and web support
game engine built on top ofMaintainers
- Loading (@load1n9)
- CarrotzRule (@carrotzrule123)
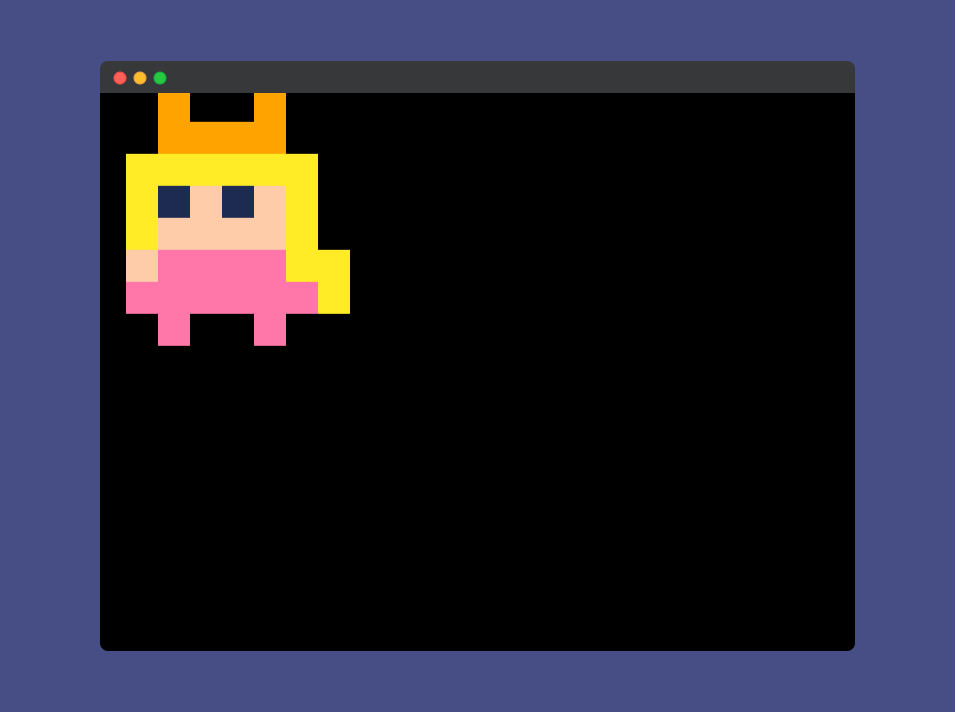
Running In the Browser
Usage
moving squares
import { World, Scene, Rectangle } from 'https://deno.land/x/caviar/mod.ts';
class Game extends Scene {
public test = new Rectangle(0, 0, 100, 100, "#00ff00");
public test2 = new Rectangle(0, 0, 100, 100, "#00ff00");
public setup() {
this.addChild(this.test);
this.addChild(this.test2);
}
public update() {
this.test.setX(this.test.x + 5);
this.test2.setX(this.test2.x + 2);
}
}
const test = new World({
title: "test",
width: 800,
height: 600,
resizable: true,
}, [Game]);
await test.start();
perlin noise
import { World, Scene, Group, Rectangle } from 'https://deno.land/x/caviar/mod.ts';
import { PerlinNoise } from "https://deno.land/x/caviar/src/utils/mod.ts";
class Game extends Scene {
public test: any;
public chunkSize = 16;
public tileSize = 16;
public group: Group | undefined;
public setup() {
this.group = new Group(this, 0,0);
this.world.loadPlugin('perlin', PerlinNoise);
this.test = this.world.usePlugin('perlin');
this.test.setSeed(0);
for (let x = -40; x < this.chunkSize; x++) {
for (let y = -40; y < this.chunkSize; y++) {
const tileX = (1 * (this.chunkSize * this.tileSize)) + (x * this.tileSize);
const tileY = (1 * (this.chunkSize * this.tileSize)) + (y * this.tileSize);
const perlinValue = this.test.perlin2(tileX / 100, tileY / 100);
if (perlinValue < 0.2) {
this.group.addChild(new Rectangle(tileX, tileY, this.tileSize, this.tileSize, '#ff0000'));
}
else if (perlinValue >= 0.2 && perlinValue < 0.3) {
this.group.addChild(new Rectangle(tileX, tileY, this.tileSize, this.tileSize, '#00ff00'));
}
else if (perlinValue >= 0.3) {
this.group.addChild(new Rectangle(tileX, tileY, this.tileSize, this.tileSize, '#0000ff'));
}
}
}
this.addChild(this.group);
}
public update() {
}
}
const test = new World({
title: "test",
width: 800,
height: 600,
resizable: true,
}, [Game]);
await test.start();
pixel texture
import { Keys, PICO8, TextureSprite, Scene, World } from 'https://deno.land/x/caviar/mod.ts';
import type { KeyEvent, MouseDownEvent } from 'https://deno.land/x/caviar/mod.ts';
class Game extends Scene {
public test = new TextureSprite(this, 10, 10, {
data: [
"..9..9..",
"..9999..",
".AAAAAA.",
".A1F1FA.",
".AFFFFA.",
".FEEEEAA",
".EEEEEEA",
"..E..E..",
],
pixelWidth: 32,
pixelHeight: 32,
palette: PICO8,
});
public setup() {
this.addChild(this.test);
}
public draw() {
}
public keyDown(key: KeyEvent) {
switch (key.keycode) {
case Keys.ARROWUP: {
this.test.setY(this.test.y - 10);
break;
}
case Keys.ARROWDOWN: {
this.test.setY(this.test.y + 10);
break;
}
case Keys.ARROWLEFT: {
this.test.setX(this.test.x - 10);
break;
}
case Keys.ARROWRIGHT: {
this.test.setX(this.test.x + 10);
break;
}
}
}
}
const test = new World({
title: "test",
width: 800,
height: 600,
centered: true,
fullscreen: false,
hidden: false,
resizable: true,
minimized: false,
maximized: false,
flags: null,
}, [Game]);
await test.start();
License
MIT
Tools
- Caviar CLI cli tool to generate caviar projects