- v5.2.0Latest
- v5.1.0
- v5.0.0
- v4.14.1
- v4.14.0
- v4.13.2
- v4.13.2
- v4.13.1
- v4.13.1
- v4.13.0
- v4.12.0
- v4.11.0
- v4.10.0
- v4.9.0
- v4.8.0
- v4.7.1
- v4.6.2
- v4.6.1
- v4.6.0
- v4.5.0
- v4.4.2
- v4.4.2
- v4.4.1
- v4.4.0
- v4.3.2
- v4.3.1
- v4.3.0
- v4.2.5
- v4.2.4
- v4.2.3
- v4.2.2
- v4.2.1
- v4.1.3
- v4.1.2
- v4.1.1
- v4.1.0
- v4.0.2
- v4.0.1
- v4.0.0
- v3.1.0
- v3.0.0
- v2.2.1
- v2.2.0
- v2.1.1
- v2.1.0
- v2.0.0
- v1.9.1
- v1.9.0
- v1.8.3
- v1.8.2
- v1.8.1
- v1.8.0
- v1.7.1
- v1.7.0
- v1.6.3
- v1.6.2
- v1.6.1
- v1.6.0
- v1.5.0
- v1.4.0
- v1.3.0
- v1.2.0
- v1.1.4
- v1.1.3
- v1.1.2
Convert
The smallest & fastest library for really easy, totally type-safe unit conversions in TypeScript & JavaScript.
yarn add convert
# or
npm install convert
Features
- Full build time and runtime checks of conversions
- Using a web framework like Next.js or Nuxt.js? You get 0-cost build-time conversions. Convert is totally side-effect free, so conversions will be precalculated at build-time, so absolutely zero conversion code is sent to clients!
- Works in browsers and Node.js (UMD, ESModules, and CommonJS builds provided)
- Out of the box ES3 backwards-compatibility (works since Node.js 0.9.1, probably earlier)
- 0 dependencies
- Supports bigints if you pass something with
typeof
bigint
Usage
API documentation for the latest version is generated and available online.
// ESM:
import convert from 'convert';
// CJS:
const {convert} = require('convert');
// 360 seconds into minutes
convert(360).from('seconds').to('minutes');
// -> 6
// BigInt support
convert(20n).from('hours').to('minutes');
// -> 1200n
// We also do length, data, volume, mass, temperature, and more
convert(5).from('kilometers').to('nautical miles');
convert(12).from('pounds').to('ounces');
convert(64).from('bytes').to('KiB');
convert(10).from('atmospheres').to('kPa');
convert(451).from('fahrenheit').to('celsius');
Converting many units
import {convertMany} from 'convert';
const {convertMany} = require('convert');
// Convert 1 day and 8 hours into ms
convertMany('1d8h').to('ms');
Contributing
Below is a list of commands you will probably find useful.
yarn start
Runs the project in development/watch mode. Your project will be rebuilt upon changes. TSDX has a special logger for you convenience. Error messages are pretty printed and formatted for compatibility VS Code’s Problems tab.
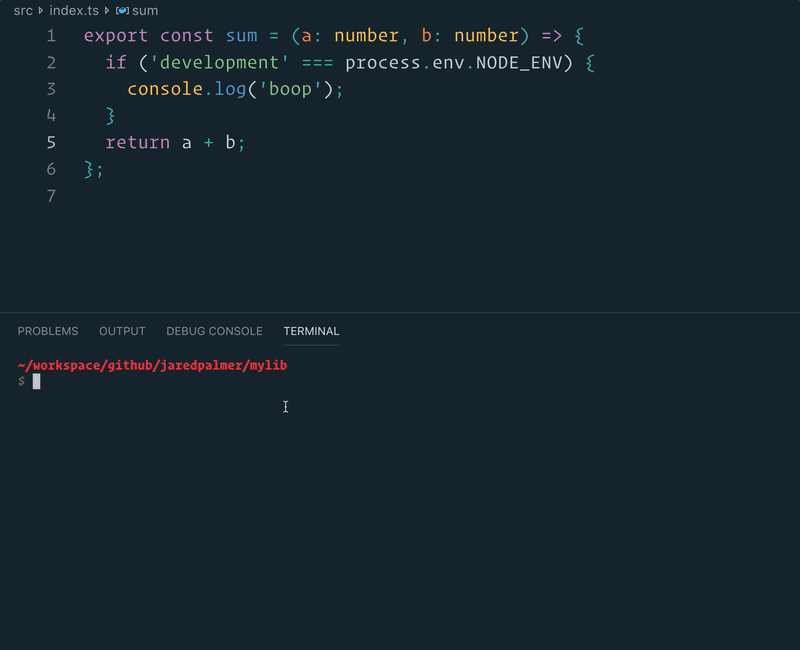
Your library will be rebuilt if you make edits.
yarn build
Bundles the package to the dist
folder.
The package is optimized and bundled with Rollup into multiple formats (CommonJS, UMD, and ES Module).
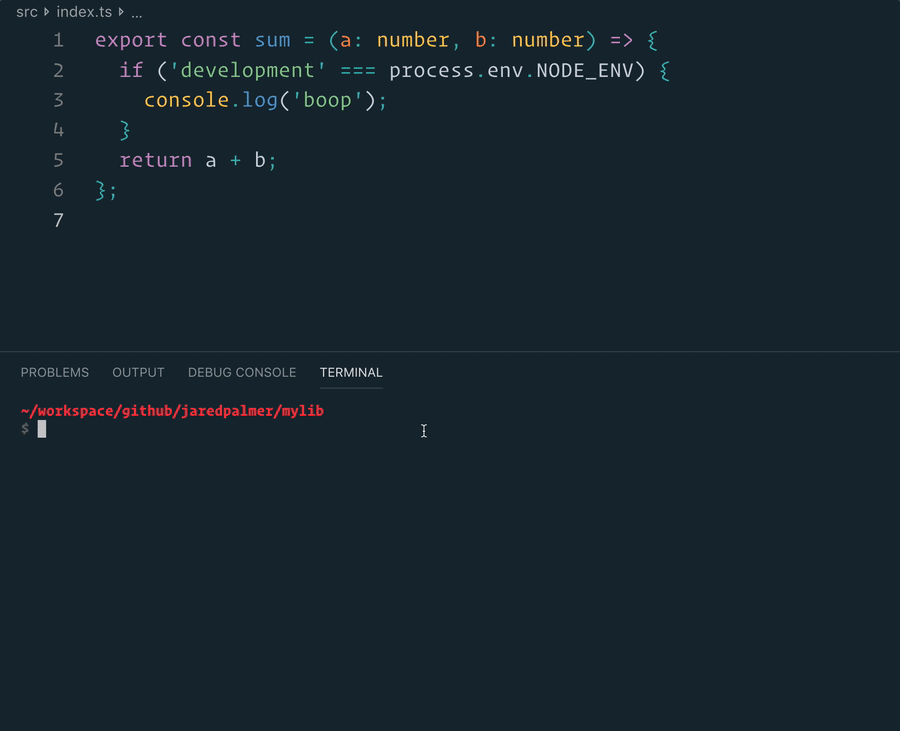
yarn test
Runs the test watcher (Jest) in an interactive mode. By default, runs tests related to files changed since the last commit.
Alternatives
All of them are bad because they aren’t as small and are slower than convert. Benchmarks of popular unit conversion libraries, including convert are available here. Convert is the fastest, taking less than a microsecond for most conversions (that’s a little bit under 2 million per second).
Thanks
Big thanks to @Jdender, @TheAkio, @iCrawl, @p7g, @aequasi, @aetheryx, and the TypeScript Discord server for their help in getting the typesafety working.
Thanks to @MicroDroid for fixing temperature conversion.