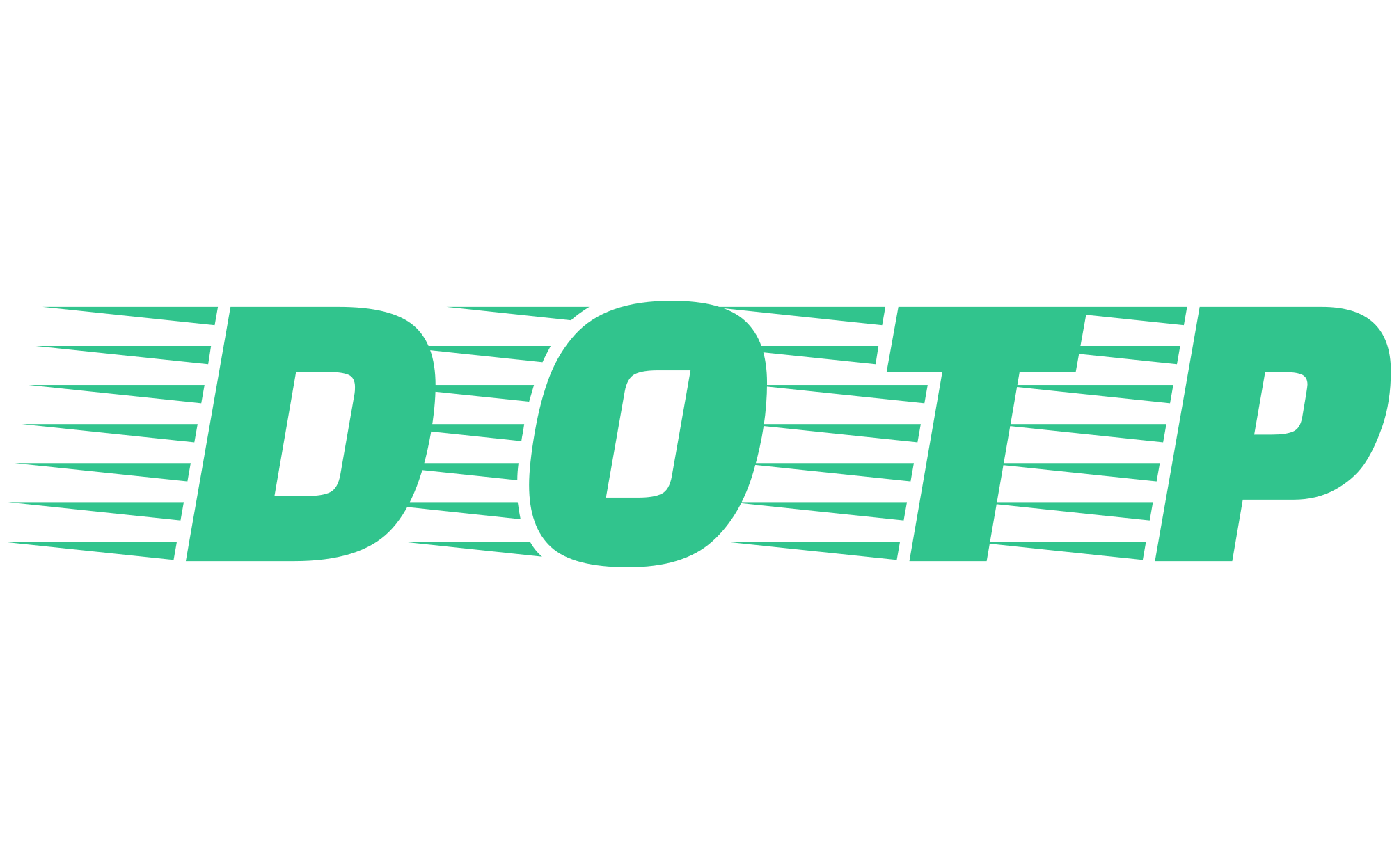
Deno One-Time Password
An implementation of HOTP (RFC 4226) and TOTP (RFC 6238) in Deno. And a little bit extra.
About
The purpose of dotp is to provide a stable and well-tested OTP library for use with Deno. The implementation aims to follow the relevant RFC documents to the letter, and to provide a developer friendly API for the features listed below.
- Create cryptographically random and secure OTP secrets
- Create hash and time based OTP tokens.
Usage
Generate Hash-based OTP
import {
createHashToken,
createMovingFactorFromNumber,
createRandomSecretKey,
} from "https://deno.land/x/dotp/mod.ts";
const secretKey = createRandomSecretKey();
const token = createHashToken(secretKey, createMovingFactorFromNumber(1));
Documentation
For a quick idea on how the module works, see the Usage section above.
API
Please visit our Documentation for details on the module API.
Examples
Go to our examples directory to learn even more.
Things to Know
HMAC-Based One-Time Password
The basis for the HMAC-Based One-Time Password (“HOTP”) algorithm is the key K
and the moving factor C
.
We compute a HMAC (hash-based message authentication code, see
RFC 2104) value from a secret key K
and and a moving factor C
using SHA-1 as the hash function. This gives us 20 bytes of data.
Then, we dynamically truncate the value. That is to say, we extract a number from the HMAC value, and then we use that value to truncate the HMAC value itself, which gives us the HOTP value.
And to put it simpler-ish. We use a large randomized number, we take a part of that number, a smaller number, and use that as the basis for slicing the big number into another number. Sometimes that final number has fewer digits than desired, so we pad it with zeros.
Time-Based One-Time Password
In order to compute a Time-Based One-Time-Password (“TOTP”) we need to use HOTP
and an agreed upon time period (technically referred to as the time step), in
seconds, which is used to compute our moving factor C
based on the number of
times our time step has happened since the beginning of the UNIX epoch.
This is how TOTP based tokens change over time, in many cases with a time step of 30 seconds.
Want to see for your self? If you have an authenticator application, you can see the token expire and a new token be generated. See how once a token expires, you are either halfway through the current minute or just starting the next.
Contributing
We do not accept contributions. Please see our ideas 💡 in the Discussions to discuss any potential ideas or improvements you may have for the project.
License
Distributed under the MIT License. See LICENSE.txt for more information.
Contact
Christoffer Hallas
hallas@libewd.com
@badgerhallas