jssm 5.100.0
Easy. Small. Fast. TS, es6, es5. Node, Browser. 100% coverage. Property tests. Fuzz tests. Language tests for a dozen languages and emoji. Easy to share online. Easy to embed.
Readable, useful state machines as one-liner strings.
5,071 tests, run 5,962 times.
- 5,062 specs with 100.0% coverage.
- 9 fuzz tests with 12.4% coverage.
With 3,007 lines, thatās about 1.7 tests per line, or 2.0 generated tests per line.
Meet your new state machine library.
TRY THE LIVE EDITOR
Discord community - Documentation - Issue tracker - CI build history
Wouldnāt it be nice if your TypeScript and Javascript state machines were simple and readable one-liners?
import { sm } from 'jssm';
const TrafficLight = sm`Red -> Green -> Yellow -> Red;`;
Wouldnāt it be great if they were easy to work with?
const log = s => console.log(s);
log( TrafficLight.state() ); // 'Red'
Machine.transition('Green'); // true
log( TrafficLight.state() ); // 'Green'
What if the notation supported action names easily?
const TLWA = sm`Red 'next' -> Green 'next' -> Yellow 'next' -> Red;`; // TLWA = Traffic Light With Actions
log( TLWA.state() ); // 'Red'
TLWA.action('next'); // true
log( TLWA.state() ); // 'Green'
TLWA.action('next'); // true
log( TLWA.state() ); // 'Yellow'
TLWA.action('next'); // true
log( TLWA.state() ); // 'Red'
What if integration with the outside was straightforward?
const MTL = sm`Red 'next' -> Green 'next' -> Yellow 'next' -> Red;` // MTL = More Traffic Lights
.hook('Red', 'Green', () => log('GO GO GO') ) // node will jump the gun when you hit return, though
.hook_entry('Red', () => log('STOP') ); // so put it on one line in node
log( MTL.state() ); // 'Red'
MTL.action('next'); // true, console logs 'GO GO GO'
log( MTL.state() ); // 'Green'
MTL.action('next'); // true
log( MTL.state() ); // 'Yellow'
MTL.action('next'); // true, console logs 'STOP'
log( MTL.state() ); // 'Red'
What if the machine followed JS standards, and distinguished refusals as false
from mistakes as throw
n?
const ATL = sm`Red -> Green -> Yellow -> Red;`; // ATL = Another Traffic Light
log( ATL.state() ); // 'Red' - uses 1st state unless told otherwise
ATL.transition('Yellow'); // false (Yellow isn't allowed from Red)
ATL.transition('Blue'); // throws (Blue isn't a state at all)
What if there were easy convenience notations for lists, and for designating main-path =>
vs available path ->
vs
only-when-forced ~>
?
const TrafficLightWithOff = sm`
Red => Green => Yellow => Red;
[Red Yellow Green] ~> Off -> Red;
`;
What if that were easy to render visually?
const TrafficLightWithOff = sm`
Red => Green => Yellow => Red;
[Red Yellow Green] ~> Off -> Red;
`;
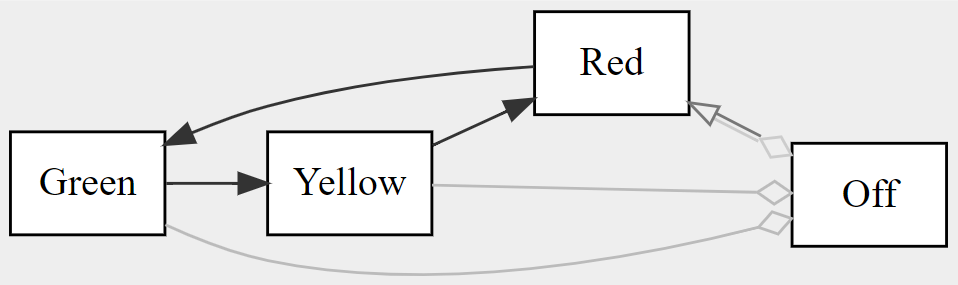
What if that were easy to render visually, with styling, in PNG, JPEG, or SVG?
const TrafficLightWithOff = sm`
Red => Green => Yellow => Red;
[Red Yellow Green] ~> Off -> Red;
flow: left;
state Red : { background-color: pink; corners: rounded; };
state Yellow : { background-color: lightyellow; corners: rounded; };
state Green : { background-color: lightgreen; corners: rounded; };
state Off : {
background-color : steelblue;
text-color : white;
shape : octagon;
linestyle : dashed;
};
`;
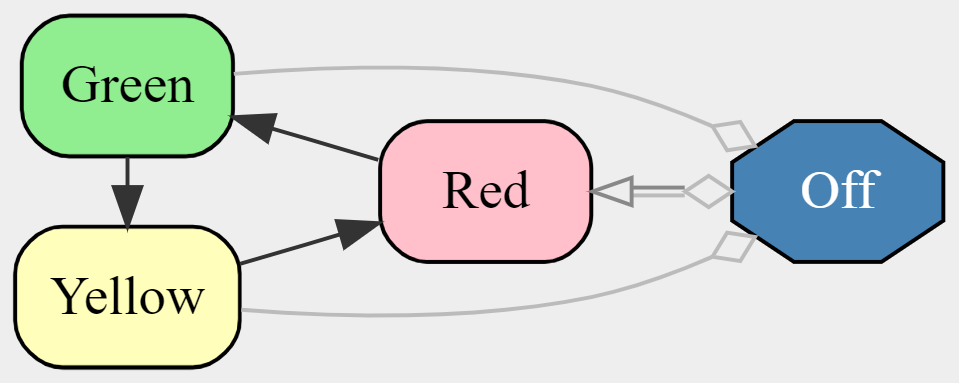
What if the machine was lighting fast, able to do tens of millions of transitions per second?

- What if the machine and language had extensive 100% test coverage with thousands of cases?
- What if the machine gave extensive Typescript introspection support?
- What if the machine had been around and active since May 2017?
- What if the machine was MIT licensed, end to end?
But, above all else:
What if it was easy?
Introducing JSSM
Meet JSSM: the Javascript State Machine.
State machines can make your code cleaner, safer, and more trustworthy.
And, with the right language, state machines can be easy and fun.
What is JSSM?
JSSM is a Javascript state machine implementing Finite State Language, with a terse DSL and a simple API. 100% test coverage; typed with Flowtype. MIT licensed.
The NPM package includes pure es6
, a cjs es5
bundle, and .d.ts
typings. The repository includes the original typescript, the bundle, the es6, documentation, tests, tutorials, and so on.
Visualize with jssm-viz, or at the command line with jssm-viz-cli.
Language test cases for Belorussian, English, German, Hebrew, Italian, Russian, Spanish, Ukrainian, and Emoji. Please help to make sure that your language is well handled!
TL;DR
Specify finite state machines with a brief syntax. Run them; theyāre fast. Make mistakes; theyāre strict. Derive charts. Save and load states, and histories. Make machine factories to churn out dozens or thousands of instances. Impress friends and loved ones. Cure corns and callouses.
Red 'Proceed' -> Green 'Proceed' -> Yellow 'Proceed' -> Red;
This will produce the following FSM (graphed with jssm-viz):
Youāll build an executable state machine.
Why
As usual, a valid question.
Why state machines
State machines are a method of making your software better able to prevent illegal states. Similar to type systems, SQL constraints, and linters, state machines are a way to teach the software to catch mistakes in ways you define, to help lead to better software.
The major mechanism of a state machine is to define states
, the transitions
between them, and sometimes associated
data
and other niceties. The minor mechanism of state machines is to attach actions
to the transitions, such that
the state machine can partially run itself.
So, to look at the same traffic light as above, youāll notice some things.
- A sufficiently smart implementation will know that itās okay for
Green
to switch toYellow
, but not toRed
- A sufficiently smart implementation knows thereās no such thing as
Blue
- A sufficiently smart implementation knows that when in
Green
, to be told toProceed
means to go toYellow
, but when inYellow
, it means to go toRed
instead
Along with other common sense things, a good state machine implementation can help eliminate large classes of error in software. State machines are often applied when the stakes on having things correct are high.
Why this implementation
Brevity.
High quality testing. JSSM has 100% coverage, and has partial stochastic test coverage.
Feature parity, especially around the DSL and data control.
Data integrity. JSSM allows a much stricter form of state machine than is common, with a relatively low performance and storage overhead. It also offers an extremely terse domain specific language (though it does not require said DSL) to produce state machines in otherwise comparatively tiny and easily read code.
Quick Start
A state machine in
JSSM
is defined in one of two ways: through the DSL, or through a datastructure.
So yeah, letās start by getting some terminology out of the way, and then we can go right back to that impenetrable sentence, so that itāll make sense.
Quick Terminology
Finite state machines have been around forever, are used by everyone, and are hugely important. As a result, the terminology is a mess, is in conflict, and is very poorly chosen, in accordince with everything-is-horrible law.
This section describes the terminology as used by this library. The author has done his best to choose a terminology that matches common use and will be familiar to most. Conflicts are explained in the following section, to keep this simple.
For this quick overview, weāll define six basic concepts:
Finite state machine
sMachine
sState
sCurrent state
Transition
sAction
s
Thereās other stuff, of course, but these five are enough to wrap your head around finite state machine
s.
Basic concepts
This is a trivial traffic light FSM
, with three states, three transitions, and one action:
Red 'Proceed' -> Green 'Proceed' -> Yellow 'Proceed' -> Red;
Letās review its pieces.
finite state machine
s- A
finite state machine
(orFSM
) is a collection ofstate
s, and rules about how you cantransition
between thestate
s. - We tend to refer to a design for a machine as āan
FSM
.ā - In this example, the traffic lightās structure is āa traffic light
FSM
.ā
- A
state
sFSM
s always have at least onestate
, and nearly always manystate
s- In this example,
- the
state
s are Red, Yellow, and Green - Something made from this
FSM
will only ever be one of those colors - not, say, Blue
- the
machine
s- Single instances of an
FSM
are referred to as amachine
- We might have a thousand instances of the traffic light designed above
- We would say āMy intersection has four
machines
of the standard three color lightFSM
.ā
- Single instances of an
current state
- A
machine
has acurrent state
, though anFSM
does not- āThis specific traffic light is currently Redā
- Traffic lights in general do not have a current color, only specific lights
FSM
s do not have a current state, only specificmachine
s- A given
machine
will always have exactly onestate
- never multiple, never none
- A
transitions
FSM
s nearly always havetransition
s- Transitions govern whether a
state
may be reached from anotherstate
- This restriction is much of the value of
FSM
s
- This restriction is much of the value of
- In this example,
- the
transition
s are- Green ā Yellow
- Yellow ā Red
- Red ā Green
- a
machine
whosecurrent state
is Green may switch to Yellow, because there is an appropriate transition - a
machine
whosecurrent state
is Green may not switch to Red, or to Green anew, because there is no such transition- A
machine
in Yellow which is told totransition
to Green (which isnāt legal) will know to refuse - This makes
FSM
s an effective tool for error prevention
- A
- the
actions
- Many
FSM
s haveaction
s, which represent events from the outside world. - In this example, there is only one action - Proceed
- The
action
Proceed is available from all three colors
- The
- At any time we may indicate to this light to go to its next color, without
taking the time to know what it is.
- This allows
FSM
s like the light to self-manage. - A
machine
in Yellow which is told to take theaction
Proceed will know on its own to switch itscurrent state
to Red. - This makes
FSM
s an effective tool for complexity reduction
- This allows
- Many
Those six ideas in hand - FSM
s, state
s, machine
s, current state
, transition
s, and action
s - and youāre ready
to move forwards.
One other quick definition - a DSL
, or domain specific language
, is when someone makes a language and embeds it into
a different language, for the purpose of attacking a specific job. When React
uses a precompiler to embed stuff that
looks like HTML in Javascript, thatās a DSL.
This library implements a simple language for defining finite state machine
s inside of strings. For example, this
DSL
defines that 'a -> b;'
actually means ācreate two states, create a transition between them, assign the first as
the initial stateā, et cetera. That micro-language is the DSL
that weāll be referring to a lot, coming up. This
DSL
ās parserās original name was jssm-dot
, because itās a descendant-in-spirit of an older flowcharting language
DOT, from graphviz, which is also used to make the
visualizations in jssm-viz by way of viz-js.
Enough history lesson. On with the tooling.
And now, that Quick Start we were talking about
So letās put together a trivial four-state traffic light: the three colors, plus Off. This will give us an opportunity to go over the basic facilities in the language.
At any time, you can take the code and put it into the graph explorer for an opportunity to mess with the code as you see fit.
0: Lights always have an off state
Our light will start in the Off state
, with the ability to switch to the Red state
.
Since thatās a normal, not-notable thing, weāll just make it a regular -> legal transition
.
Off -> Red;
We will give that transition
an action
, and call it TurnOn.
Off 'TurnOn' -> Red;
So far, our machine is simple:
1: Traffic lights have a three-color cycle
The main path of a traffic light is cycling from Green to Yellow, then to Red, then back again. Because
this is the main path, weāll mark these steps => main transition
s.
Off 'TurnOn' -> Red => Green => Yellow => Red;
We will give those all the same action name, Proceed, indicating ānext colorā without needing to know what weāre currently on.
Off 'TurnOn' -> Red 'Proceed' => Green 'Proceed' => Yellow 'Proceed' => Red;
Machineās still pretty simple:
2: Traffic lights can be shut down
Weād also like to be able to turn this light back off. Because thatās expected to be a rarity, weāll require that it
be a ~> forced transition
.
We could write
Off 'TurnOn' -> Red 'Proceed' => Green 'Proceed' => Yellow 'Proceed' => Red;
Red ~> Off;
Yellow ~> Off;
Green ~> Off;
But that takes a lot of space even with this short list, so, instead weāll use the array notation
Off 'TurnOn' -> Red 'Proceed' => Green 'Proceed' => Yellow 'Proceed' => Red;
[Red Yellow Green] ~> Off;
And weād like those all to have the action TurnOff, so
Off 'TurnOn' -> Red 'Proceed' => Green 'Proceed' => Yellow 'Proceed' => Red;
[Red Yellow Green] 'TurnOff' ~> Off;
Machineās still not too bad:
Letās actually use the traffic light
Thatās actually the bulk of the language. There are other little add-ons here and there, but, primarily you now know how to write a state machine.
Letās load it and use it! š
loading into node
loading into html
jssm-viz
redistribution on npm
An introduction to machine design
Letās make a state machine
for ATMs. In the process, we will use a lot of core concepts of finite state machine
s
and of fsl
, this libraryās DSL
.
Weāre going to improve on this NCSU ATM diagram that I found:
Remember, at any time, you can take the code and put it into the graph explorer for an opportunity to mess with the code as you see fit.
0: Empty machine
Weāll start with an empty machine.
EmptyWaiting 'Wait' -> EmptyWaiting;
1: Should be able to eject cards
Weāll add the ability to physically eject the userās card and reset to the empty and waiting state. Right now itāll dangle around un-used at the top, but later itāll become useful.
This is expressed as the path EjectCardAndReset -> EmptyWaiting;
EmptyWaiting 'Wait' -> EmptyWaiting;
EjectCardAndReset -> EmptyWaiting;
2: Should be able to insert cards
Weāll add the ability to physically insert a card, next. You know, the, uh, thing ATMs are pretty much for.
To get this, add the path leg EmptyWaiting 'InsertCard' -> HasCardNoAuth;
EmptyWaiting 'Wait' -> EmptyWaiting 'InsertCard' -> HasCardNoAuth;
EjectCardAndReset -> EmptyWaiting;
Notice that the new state
, HasCardNoAuth, has been rendered red. This is because it is terminal
- there is
no exit from this node currently. (EmptyAndWaiting did not render that way because it had a transition to itself.)
That will change as we go back to adding more nodes. terminal node
s are usually either mistakes or the last single
state
of a given FSM
.
3: Should be able to cancel and recover the card
Next, we should have a cancel, because the ATMās 7 key is broken, and we need our card back. Cancel will exit to the main menu, and return our card credential.
To that end, we add the path HasCardNoAuth 'CancelAuthReturnCard' -> EjectCardAndReset;
EmptyWaiting 'Wait' -> EmptyWaiting 'InsertCard' -> HasCardNoAuth;
HasCardNoAuth 'CancelAuthReturnCard' -> EjectCardAndReset;
EjectCardAndReset -> EmptyWaiting;
4: Can give the wrong PIN
Next, letās give the ability to get the password ⦠wrong. š Because we all know that one ATM that only has the wrong-PIN path, so, apparently thatās a product to someone.
When they get the PIN wrong, theyāre prompted to try again (or to cancel.)
Weāll add the path HasCardNoAuth 'WrongPIN' -> HasCardNoAuth;
EmptyWaiting 'Wait' -> EmptyWaiting 'InsertCard' -> HasCardNoAuth;
HasCardNoAuth 'CancelAuthReturnCard' -> EjectCardAndReset;
HasCardNoAuth 'WrongPIN' -> HasCardNoAuth;
EjectCardAndReset -> EmptyWaiting;
5: Can give the correct PIN
Next, letās give the ability to get the password right.
Weāll add two paths. The first gets the password right: HasCardNoAuth 'RightPIN' -> MainMenu;
The second, from our new state
MainMenu, gives people the ability to leave: MainMenu 'ExitReturnCard' -> EjectCardAndReset;
EmptyWaiting 'Wait' -> EmptyWaiting 'InsertCard' -> HasCardNoAuth;
HasCardNoAuth 'CancelAuthReturnCard' -> EjectCardAndReset;
HasCardNoAuth 'WrongPIN' -> HasCardNoAuth;
HasCardNoAuth 'RightPIN' -> MainMenu;
MainMenu 'ExitReturnCard' -> EjectCardAndReset;
EjectCardAndReset -> EmptyWaiting;
6: Can check balance from main menu
Hooray, now weāre getting somewhere.
Letās add the ability to check your balance. First pick that from the main menu, then pick which account to see the balance of, then youāre shown a screen with the information you requested; then go back to the main menu.
Thatās MainMenu 'CheckBalance' -> PickAccount -> DisplayBalance -> MainMenu;
.
EmptyWaiting 'Wait' -> EmptyWaiting 'InsertCard' -> HasCardNoAuth;
HasCardNoAuth 'CancelAuthReturnCard' -> EjectCardAndReset;
HasCardNoAuth 'WrongPIN' -> HasCardNoAuth;
HasCardNoAuth 'RightPIN' -> MainMenu;
MainMenu 'ExitReturnCard' -> EjectCardAndReset;
MainMenu 'CheckBalance' -> PickAccount -> DisplayBalance -> MainMenu;
EjectCardAndReset -> EmptyWaiting;
7: Can deposit money from main menu
Letās add something difficult. Their state machine just proceeds assuming everything is okay.
To desposit money:
- Accept physical money
- If accept failed (eg door jammed,) reject physical object, go to main menu
- If accept succeeded, ask human expected value
- Pick an account this should go into
- Contact bank. Request to credit for theoretical physical money.
- Three results: yes, no, offer-after-audit.
- If no, reject physical object, go to main menu.
- If yes, consume physical object, tell user consumed, go to main menu
- If offer-after-audit, ask human what to do
- if human-yes, consume physical object, tell user consumed, go to main menu
- if human-no, reject physical object, go to main menu
Writing this out in code is not only generally longer than the text form, but also error prone and hard to maintain.
⦠or thereās the FSM
DSL
, which is usually as-brief-as the text, and frequently both briefer and more explicit.
- Rules 1-2:
MainMenu 'AcceptDeposit' -> TentativeAcceptMoney 'AcceptFail' -> RejectPhysicalMoney -> MainMenu;
- Rules 3-6:
TentativeAcceptMoney 'AcceptSucceed' -> PickDepositAccount -> RequestValue 'TellBank' -> BankResponse;
- Rule 7:
BankResponse 'BankNo' -> RejectPhysicalMoney;
- Rule 8:
BankResponse 'BankYes' -> ConsumeMoney -> NotifyConsumed -> MainMenu;
- Rules 9-10:
BankResponse 'BankAudit' -> BankAuditOffer 'HumanAcceptAudit' -> ConsumeMoney;
- Rule 11:
BankAuditOffer 'HumanRejectAudit' -> RejectPhysicalMoney;
Or, as a block,
MainMenu 'AcceptDeposit' -> TentativeAcceptMoney;
TentativeAcceptMoney 'AcceptFail' -> RejectPhysicalMoney -> MainMenu;
TentativeAcceptMoney 'AcceptSucceed' -> PickDepositAccount -> RequestValue 'TellBank' -> BankResponse;
BankResponse 'BankNo' -> RejectPhysicalMoney;
BankResponse 'BankYes' -> ConsumeMoney -> NotifyConsumed -> MainMenu;
BankResponse 'BankAudit' -> BankAuditOffer 'HumanAcceptAudit' -> ConsumeMoney;
BankAuditOffer 'HumanRejectAudit' -> RejectPhysicalMoney;
Which leaves us with the total code
EmptyWaiting 'Wait' -> EmptyWaiting 'InsertCard' -> HasCardNoAuth;
HasCardNoAuth 'CancelAuthReturnCard' -> EjectCardAndReset;
HasCardNoAuth 'WrongPIN' -> HasCardNoAuth;
HasCardNoAuth 'RightPIN' -> MainMenu;
MainMenu 'AcceptDeposit' -> TentativeAcceptMoney;
MainMenu 'ExitReturnCard' -> EjectCardAndReset;
MainMenu 'CheckBalance' -> PickCheckBalanceAccount -> DisplayBalance -> MainMenu;
TentativeAcceptMoney 'AcceptFail' -> RejectPhysicalMoney -> MainMenu;
TentativeAcceptMoney 'AcceptSucceed' -> PickDepositAccount -> RequestValue 'TellBank' -> BankResponse;
BankResponse 'BankNo' -> RejectPhysicalMoney;
BankResponse 'BankYes' -> ConsumeMoney -> NotifyConsumed -> MainMenu;
BankResponse 'BankAudit' -> BankAuditOffer 'HumanAcceptAudit' -> ConsumeMoney;
BankAuditOffer 'HumanRejectAudit' -> RejectPhysicalMoney;
EjectCardAndReset -> EmptyWaiting;
8: Can withdraw money from main menu
Letās also be able to take money from the machine. After this, weāll move on, since our example is pretty squarely made by now.
- Pick a withdrawl account, or cancel to the main menu
- Shown a balance, pick a withdrawl amount, or cancel to acct picker
- Is the withdrawl account too high? If so go to 2
- Does the machine actually have the money? If not go to 2
- Otherwise confirm intent w/ human
- Attempt to post the transaction.
- If fail, display reason and go to 1
- If succeed, dispense money and go to main menu
- Rules 1-3:
MainMenu -> PickWithdrawlAccount -> PickAmount -> AcctHasMoney? 'TooHighForAcct' -> PickWithdrawlAccount;
- Rule 4:
AcctHasMoney? -> MachineHasMoney? 'MachineLowOnCash' -> PickAmount;
- Rule 5:
MachineHasMoney? -> ConfirmWithdrawWithHuman 'MakeChanges' -> PickWithdrawlAmount;
- Rule 6:
ConfirmWithdrawWithHuman 'PostWithdrawl' -> BankWithdrawlResponse;
- Rule 7:
BankWithdrawlResponse 'WithdrawlFailure' -> WithdrawlFailureExplanation -> PickWithdrawlAccount;
- Rule 8:
BankWithdrawlResponse 'WithdrawlSuccess' -> DispenseMoney -> MainMenu;
Rule 1 canceller: PickWithdrawlAccount 'CancelWithdrawl' -> MainMenu;
Rule 2 canceller: PickWithdrawlAmount 'SwitchAccounts' -> PickWithdrawlAccount;
Or as a whole, weāre adding
MainMenu -> PickWithdrawlAccount -> PickAmount -> AcctHasMoney? 'TooHighForAcct' -> PickWithdrawlAccount;
AcctHasMoney? -> MachineHasMoney? 'MachineLowOnCash' -> PickAmount;
MachineHasMoney? -> ConfirmWithdrawWithHuman 'MakeChanges' -> PickWithdrawlAmount;
ConfirmWithdrawWithHuman 'PostWithdrawl' -> BankWithdrawlResponse;
BankWithdrawlResponse 'WithdrawlFailure' -> WithdrawlFailureExplanation -> PickWithdrawlAccount;
BankWithdrawlResponse 'WithdrawlSuccess' -> DispenseMoney -> MainMenu;
PickWithdrawlAccount 'CancelWithdrawl' -> MainMenu;
PickWithdrawlAmount 'SwitchAccounts' -> PickWithdrawlAccount;
Which leaves us with
EmptyWaiting 'Wait' -> EmptyWaiting 'InsertCard' -> HasCardNoAuth;
HasCardNoAuth 'CancelAuthReturnCard' -> EjectCardAndReset;
HasCardNoAuth 'WrongPIN' -> HasCardNoAuth;
HasCardNoAuth 'RightPIN' -> MainMenu;
MainMenu 'AcceptDeposit' -> TentativeAcceptMoney;
MainMenu 'ExitReturnCard' -> EjectCardAndReset;
MainMenu 'CheckBalance' -> PickCheckBalanceAccount -> DisplayBalance -> MainMenu;
TentativeAcceptMoney 'AcceptFail' -> RejectPhysicalMoney -> MainMenu;
TentativeAcceptMoney 'AcceptSucceed' -> PickDepositAccount -> RequestValue 'TellBank' -> BankResponse;
BankResponse 'BankNo' -> RejectPhysicalMoney;
BankResponse 'BankYes' -> ConsumeMoney -> NotifyConsumed -> MainMenu;
BankResponse 'BankAudit' -> BankAuditOffer 'HumanAcceptAudit' -> ConsumeMoney;
BankAuditOffer 'HumanRejectAudit' -> RejectPhysicalMoney;
MainMenu -> PickWithdrawlAccount -> PickAmount -> AcctHasMoney? 'TooHighForAcct' -> PickWithdrawlAccount;
AcctHasMoney? -> MachineHasMoney? 'MachineLowOnCash' -> PickAmount;
MachineHasMoney? -> ConfirmWithdrawWithHuman 'MakeChanges' -> PickWithdrawlAmount;
ConfirmWithdrawWithHuman 'PostWithdrawl' -> BankWithdrawlResponse;
BankWithdrawlResponse 'WithdrawlFailure' -> WithdrawlFailureExplanation -> PickWithdrawlAccount;
BankWithdrawlResponse 'WithdrawlSuccess' -> DispenseMoney -> MainMenu;
PickWithdrawlAccount 'CancelWithdrawl' -> MainMenu;
PickWithdrawlAmount 'SwitchAccounts' -> PickWithdrawlAccount;
EjectCardAndReset -> EmptyWaiting;
As you can see, building up even very complex state machines is actually relatively straightforward, in a short amount of time.
Features
DSL
States
Transitions
Cycles
Stripes
Named Ordered Lists
Atoms
Strings
Arrow types
Unicode representations
Node declarations
All the styling bullshit
Named edges
URL callouts
The 9 or whatever directives
How to publish a machine
Legal, main, and forced
Validators
State history
Automatic visualization
How to think in state machines
Example Machines
Door lock
Traffic lights
Basic three-state
RYG, Off, Flash-red, Flash-yellow
RYG, Off, Flash-red, Flash-yellow, Green-left, Yellow-left
Heirarchal intersection
ATM
HTTP
Better HTTP
TCP
Coin-op vending machine (data)
Video games
Pac-man Ghost (sensors)
Weather (probabilistics)
Roguelike monster (interface satisfaction)
Candy crush clone game flow (practical large use)
Vegas locked 21 dealer behavior
React SPA website (practical large use)
BGP
LibGCrypt FIPS mode FSM
How to debug
How to publish
Itās really quite simple.
- Make a github repository.
- Put your code in a file inside, with the extension
.fsl
- Make sure your code contains a
machine_name
Once done, your work should show up here.
Notation Comparison
Their notations, one by one
Apples to Apples - Traffic Light
Other state machines
There are a lot of state machine impls for JS, many quite a bit more mature than this one. Here are some options:
- Finity š®
- Stately.js
- machina.js
- Pastafarian
- Henderson
- fsm-as-promised
- state-machine
- mood
- FSM Workbench
- SimpleStateMachine
- shime/micro-machine
- soveran/micromachine (ruby)
- fabiospampinato/FSM
- HQarroum/FSM
- Finite-State-Automata
- finite-state-machine
- nfm
And some similar stuff:
- redux-machine
- ember-fsm
- State machine cat
- Workty š®
- sam-simpler
- event_chain
- DRAKON
- Yakindu Statechart Tools
- GraphViz
- Viz.js, which we use
Thanks
JSSM and FSL have had a lot of help.
Internationalization
- Mykhaylo Les provided three translation test cases (Ukrainian, Belarussian, and Russian,) and the corresponding Traffic Light translations (also Ukrainian, Belarussian, and Russian.)
- Tanvir Islam provided the Bengali test case, translated the Traffic Light to Bengali, and published the first non-English
FSL
machine, in Bengali. - Francisco Junior provided the Portuguese test case and translated the Traffic Light to Portuguese
- Jeff Katz provided the German test case.
- Alex Cresswell provdied the Spanish test case
- Dvir Cohen provided the Hebrew test case.
- David de la PeƱa provided the French test case
If Iāve overlooked you, please let me know.
If youād like to help, itās straightforward.
- Easy mode: open a PR with this file translated into your language
- Extra mile: create a new repo containing this file translated
Code and Language
Vat Raghavan has participated extensively in language discussion and implemented several features.
Forest Belton has provided guidance, bugfixes, parser and language commentary.
Jordan Harbrand suggested two interesting features and provided strong feedback on the initial tutorial draft.
The biggest thanks must go to Michael Morgan, who has debated significant sections of
the notation, invented several concepts and operators, helped with the parser, with system nomenclature, for having published
the first not-by-me FSL
machine, for encouragement, and generally just for having been as interested as he has been.