A collection of fast, scalable, low-level Deno modules for interacting with the Discord API.
Repository
Current version released
3 years ago
Space
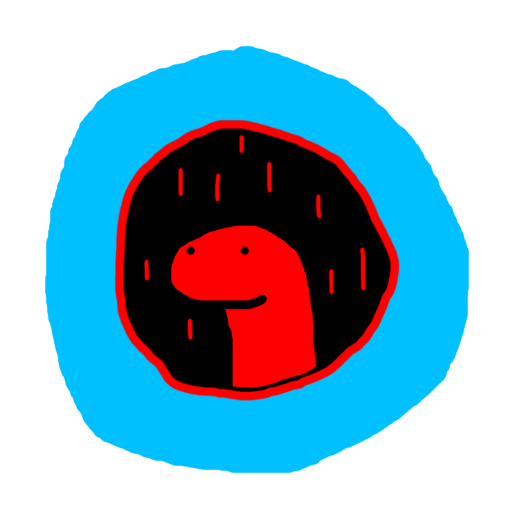
A low-level Deno module for interacting with Discord.
Features
- Secure by default. Client-side checks prevent
4xx
s from occuring. - Written purely in TypeScript to guarantee type safety.
- Standalone API modules (HTTP, interactions, websocket).
- Built-in utilities such as event logging and custom caching.
- 100% coverage over Discord’s HTTP and websocket APIs.
Install
export * from "https://deno.land/x/space@0.9.0-alpha/mod.ts";
See the release notes for all available versions.
Getting Started
Simple program to get started:
import { Client, GatewayIntentBits, onMessageCreate } from "./deps.ts";
const token = Deno.env.get("TOKEN");
const client = new Client(`Bot ${token}`);
client.connect({
intents: GatewayIntentBits.GUILD_MESSAGES,
});
for await (const [data, shard] of client.gateway.listen("MESSAGE_CREATE")) {
const message = await onMessageCreate(client, data);
if (message.content === "!ping") {
client.rest.createMessage(message.channelID, {
content: "pong!",
});
}
}
Simple interactions program:
import { InteractionResponseType, Server } from "./deps.ts";
const publicKey = Deno.env.get("PUBLIC_KEY");
const server = new Server(publicKey);
server.connect(1337);
for await (const [interaction, respond] of server.listen("COMMAND")) {
if (interaction.data.name === "ping") {
respond({
type: InteractionResponseType.ChannelMessageWithSource,
data: { content: "pong" },
});
}
}
See the documentation for reference.