Attributes
Includes Deno configuration
Repository
Current version released
11 months ago
⌨️ Tui
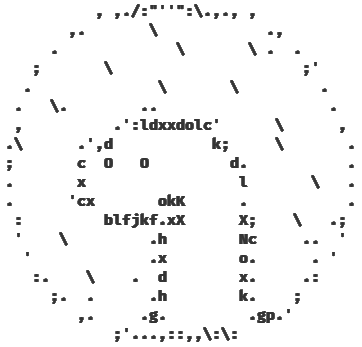
Simple Deno module that allows easy creation of Terminal User Interfaces.
🔩 Features
- 🔰 Ease of use
- 👁️🗨️ Reactivity
- 🖇️ No dependencies
- 📄 Decent documentation
- 📦 Multiple ready-to-use components
- 🎨 Styling framework agnostic
- This means you can use whatever terminal styling module you want
- 🖍️ Crayon is recommended but not imposed as it greatly integrates with Tui
- 🪶 Relatively lightweight
🖥️ OS Support
Operating system | Linux | macOS | Windows¹ | WSL |
---|---|---|---|---|
Base | ✔️ | ✔️ | ✔️ | ✔️ |
Keyboard support | ✔️ | ✔️ | ✔️ | ✔️ |
Mouse support | ✔️ | ✔️ | ✔️ | ✔️ |
Required permissions | none | none | none | none |
¹ - If unicode characters are displayed incorrectly type chcp 65001
into the console to change active console code
page to use UTF-8 encoding.
🎓 Get started
Replace {version} with relevant module versions
- Create Tui instance
import { crayon } from "https://deno.land/x/crayon@2.1.11/mod.ts";
import { Canvas, Tui } from "https://deno.land/x/tui@2.1.11/mod.ts";
const tui = new Tui({
style: crayon.bgBlack, // Make background black
refreshRate: 1000 / 60, // Run in 60FPS
});
tui.dispatch(); // Close Tui on CTRL+C
- Enable interaction using keyboard and mouse
import { handleInput, handleKeyboardControls, handleMouseControls } from "https://deno.land/x/tui@2.1.11/mod.ts";
...
handleInput(tui);
handleMouseControls(tui);
handleKeyboardControls(tui);
- Add some components
import { Button } from "https://deno.land/x/tui@2.1.11/src/components/mod.ts";
import { Signal, Computed } from "https://deno.land/x/tui@2.1.11/mod.ts";
...
// Create signal to make number automatically reactive
const number = new Signal(0);
const button = new Button({
parent: tui,
zIndex: 0,
label: {
text: new Computed(() => number.value.toString()), // cast number to string
},
theme: {
base: crayon.bgRed,
focused: crayon.bgLightRed,
active: crayon.bgYellow,
},
rectangle: {
column: 1,
row: 1,
height: 5,
width: 10,
},
});
// If button is active (pressed) make number bigger by one
button.state.when("active", (state) => {
++number.value;
});
// Listen to mousePress event
button.on("mousePress", ({ drag, movementX, movementY }) => {
if (!drag) return;
// Use peek() to get signal's value when it happens outside of Signal/Computed/Effect
const rectangle = button.rectangle.peek();
// Move button by how much mouse has moved while dragging it
rectangle.column += movementX;
rectangle.row += movementY;
});
- Run Tui
...
tui.run();
🤝 Contributing
Tui is open for any contributions.
If you feel like you can enhance this project - please open an issue and/or pull request.
Code should be well document and easy to follow what’s going on.
This project follows conventional commits spec.
If your pull request’s code can be hard to understand, please add comments to it.
📝 Licensing
This project is available under MIT License conditions.