Repository
Current version released
2 years ago
⌨️ Tui
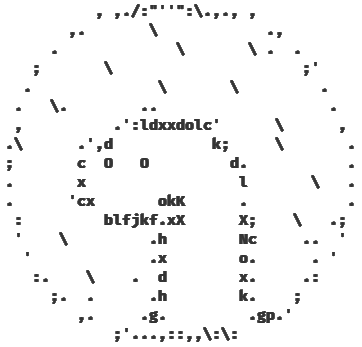
Current status: Release candidate
Tui is a simple Deno module that allows easy creation of Terminal User Interfaces.
Features
- Decent documentation
- Multiple built-in components
- Respects
NO_COLOR
env variable - Reactivity
- Simple to use
- Zero dependencies
- Relatively lightweight
🎓 Get started
- Create tui instance
import {
compileStyler,
createTui,
TuiStyler,
} from "https://deno.land/x/tui@version/mod.ts";
const tui = createTui({
// Which stdin we read inputs from
reader: Deno.stdin,
// To which stdout we render tui
writer: Deno.stdout,
// How tui should look
styler: compileStyler<TuiStyler>({
foreground: "white",
background: "black",
}),
});
- Enable handling keyboard and mouse
import {
...,
handleKeypresses,
handleKeyboardControls,
handleMouseControls
} from "https://deno.land/x/tui@version/mod.ts";
...
// Needed for both keyboard and mouse controls handlers to work
handleKeypresses(tui);
// Enable keyboard controls
handleKeyboardControls(tui);
// Enable mouse controls
handleMouseControls(tui);
- Add some components
import { ..., createButton } from "https://deno.land/x/tui@version/mod.ts";
...
const componentStyler = compileStyler<TuiStyler>({
...tui.styler,
background: "blue",
focused: {
attributes: ["bold"],
background: "green",
},
active: {
attributes: ["bold", "italic"],
foreground: "black",
background: "lightCyan",
},
});
let counter = 0;
const button = createButton(tui, {
label: {
get text() {
return String(counter);
},
align: {
vertical: "center",
horizontal: "center",
},
},
rectangle: {
column: 2,
row: 1,
width: 5,
height: 1,
},
});
// when button is activated increase counter
button.on("active", () => {
++counter;
});
- Render tui
import { ..., loopDrawing } from "https://deno.land/x/tui@version/mod.ts";
...
loopDrawing(tui);
🤝 Contributing
I’m open to any idea and criticism. Feel free to add any commits, issues and pull requests!
📝 Licensing
This project is available under MIT License conditions.